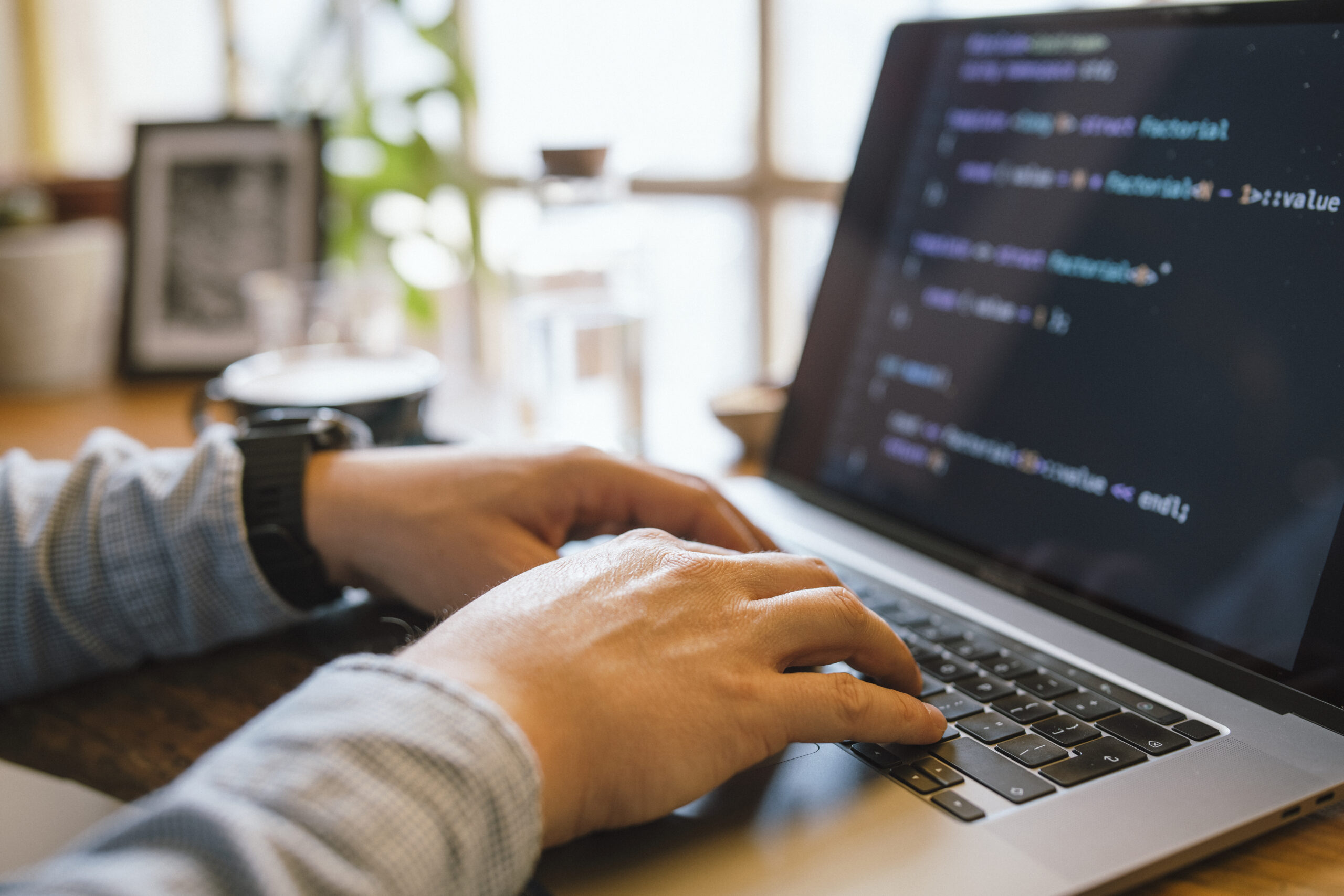
Debugging is Just about the most critical — however typically forgotten — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging abilities can conserve hours of stress and considerably transform your productiveness. Here are a number of methods to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest approaches developers can elevate their debugging skills is by mastering the resources they use each day. Whilst crafting code is just one Section of advancement, understanding the best way to interact with it successfully during execution is Similarly significant. Modern-day advancement environments come equipped with powerful debugging abilities — but several developers only scratch the floor of what these equipment can do.
Consider, for instance, an Built-in Enhancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, stage by means of code line by line, and even modify code to the fly. When utilised properly, they Permit you to observe accurately how your code behaves all through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective genuine-time effectiveness metrics, and debug JavaScript while in the browser. Mastering the console, sources, and network tabs can convert frustrating UI troubles into workable duties.
For backend or procedure-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than running processes and memory administration. Discovering these resources could possibly have a steeper Discovering curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Handle programs like Git to know code background, uncover the precise instant bugs were introduced, and isolate problematic modifications.
Finally, mastering your applications implies likely outside of default configurations and shortcuts — it’s about developing an intimate familiarity with your progress ecosystem so that when problems arise, you’re not misplaced at the hours of darkness. The greater you know your resources, the more time you are able to devote fixing the particular challenge in lieu of fumbling by the procedure.
Reproduce the situation
Among the most important — and infrequently missed — steps in effective debugging is reproducing the condition. Right before leaping to the code or building guesses, developers require to create a dependable natural environment or circumstance in which the bug reliably appears. With out reproducibility, correcting a bug results in being a video game of possibility, usually leading to wasted time and fragile code variations.
Step one in reproducing an issue is gathering just as much context as you can. Ask concerns like: What actions brought about The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The more depth you've got, the simpler it results in being to isolate the exact conditions underneath which the bug happens.
When you’ve gathered ample details, attempt to recreate the condition in your local atmosphere. This may imply inputting a similar knowledge, simulating similar consumer interactions, or mimicking system states. If The difficulty appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions associated. These exams don't just aid expose the condition but in addition stop regressions Later on.
At times, The difficulty may be setting-unique — it would transpire only on certain running units, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a step — it’s a state of mind. It needs endurance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible situation, You need to use your debugging equipment far more proficiently, take a look at opportunity fixes properly, and connect much more clearly together with your group or customers. It turns an abstract complaint right into a concrete challenge — and that’s where developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages in many cases are the most beneficial clues a developer has when a thing goes Erroneous. As an alternative to viewing them as aggravating interruptions, developers should really discover to take care of mistake messages as direct communications in the system. They normally inform you what exactly took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Start by looking at the concept diligently As well as in total. Many builders, particularly when under time force, glance at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the mistake down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or operate triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly accelerate your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to look at the context wherein the error happened. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These generally precede larger problems and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an application behaves, helping you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with being aware of what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts throughout improvement, INFO for typical situations (like prosperous start off-ups), WARN for possible issues that don’t break the applying, Mistake for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target important situations, point out changes, enter/output values, and demanding conclusion factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly important in manufacturing environments wherever stepping via code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a perfectly-imagined-out logging method, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a sort of investigation. To effectively discover and take care of bugs, developers should technique the method similar to a detective resolving a secret. This state of mind aids stop working elaborate problems into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by gathering evidence. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, acquire as much pertinent information and facts as you could without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications lately been made to the codebase? Has this problem occurred right before underneath related conditions? The objective is to slender down choices and identify potential culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if the issue persists. Similar to a detective conducting interviews, check with your code queries and let the effects direct you nearer to the reality.
Spend shut awareness to tiny details. Bugs generally conceal during the minimum expected spots—like a lacking semicolon, an off-by-1 mistake, or possibly a race condition. Be extensive and affected person, resisting the urge to patch The problem with out thoroughly comprehending it. Momentary fixes could disguise the true problem, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future concerns and enable others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering concealed challenges in complex techniques.
Produce Checks
Writing exams is one of the best tips on how to improve your debugging abilities and In general development efficiency. Exams not merely support capture bugs early and also function a safety net that gives you self esteem when earning variations to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint precisely in which and when an issue takes place.
Begin with device exams, which target specific features or modules. These modest, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glimpse, noticeably cutting down enough time invested debugging. Unit checks are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration exams and end-to-close assessments into your workflow. These enable be certain that numerous parts of your software perform together effortlessly. They’re specifically helpful for catching bugs that manifest in intricate methods with various factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Assume critically about your code. To test a element effectively, you would like to grasp its inputs, envisioned outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can target correcting the bug and view your take a look at go when The problem is solved. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the trouble—observing your display screen for several hours, seeking solution soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease disappointment, and sometimes see The problem from a new perspective.
When you're way too near to the code for also prolonged, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hrs earlier. Within this state, your Mind will become less efficient at trouble-resolving. A brief walk, a coffee crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may experience counterintuitive, Specially less than restricted deadlines, but it really truly causes quicker and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your Mind House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you anything important if you make an effort to mirror and examine what went wrong.
Begin by asking oneself a number of essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught Gustavo Woltmann coding earlier with much better methods like unit testing, code critiques, or logging? The answers often reveal blind spots within your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack concept, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important aspects of your progress journey. In any case, a lot of the greatest builders usually are not those who create fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a minute to replicate—you’ll come away a smarter, extra capable developer as a result of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is huge. It tends to make you a far more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.